🚀🌕 Code that took us to the Moon: What we can learn from Apollo 11's Source Code 🛰
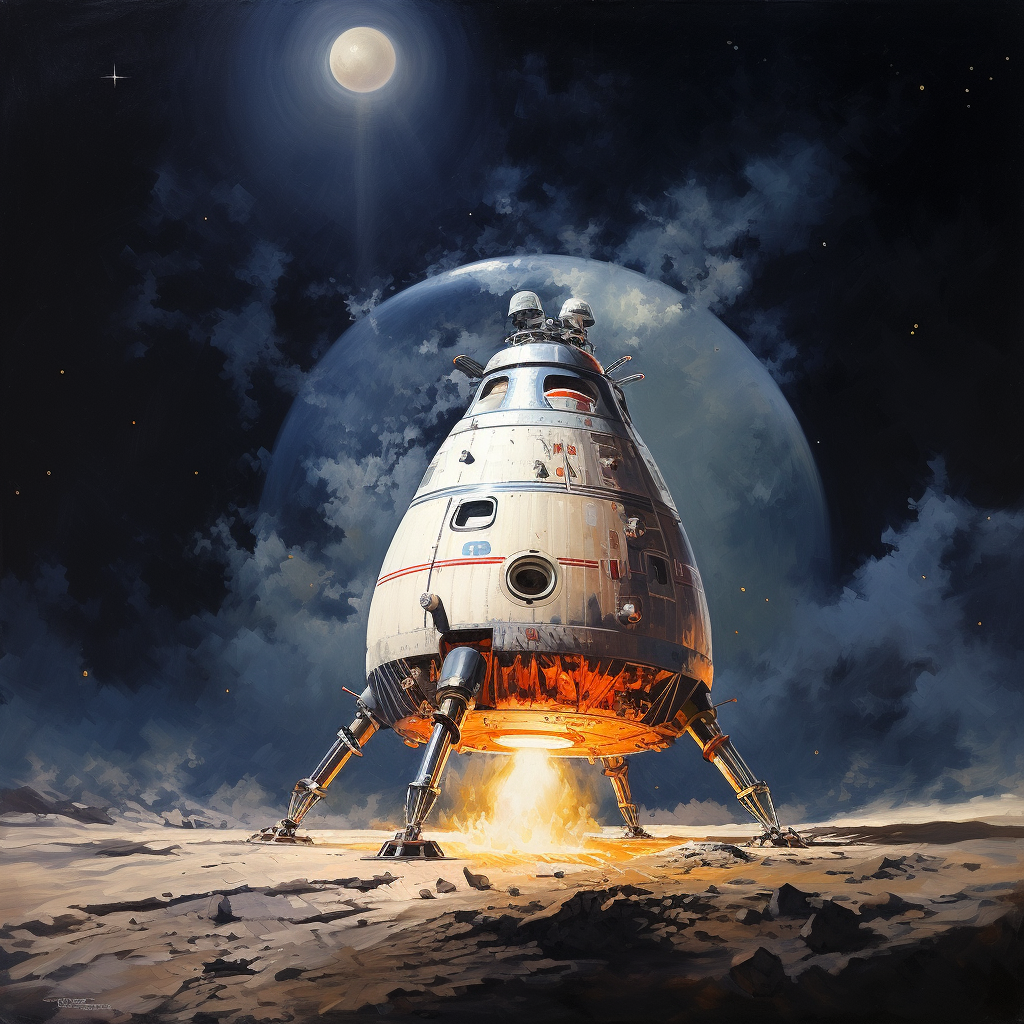
In July 1969, humanity achieved one of its most audacious technological feats - landing men on the moon. Powering this achievement was a piece of engineering brilliance known as the Apollo Guidance Computer (AGC), a system responsible for navigating the vast expanse of space to our celestial neighbour and back. Interestingly, the AGC's source code, a monument to engineering simplicity, robustness, and organisation, is publicly accessible. It provides invaluable insights into effective software engineering principles that are just as relevant today as they were over 50 years ago.
You can dig into the source code for Original Apollo 11 guidance computer (AGC) source code for Command Module (Comanche055) and Lunar Module (Luminary099). Digitised by the folks at Virtual AGC and MIT Museum in this GitHub repository. This source code has been transcribed or otherwise adapted from digitised images of a hardcopy from the MIT Museum. The digitisation was performed by Paul Fjeld, and arranged for by Deborah Douglas of the Museum. Many thanks to both.
Modularity: Organised Code for an Organised Mission
The AGC's source code is an excellent example of organising a large and complex codebase. Written in an assembly language, the code is impressively well-structured. Tasks are split into separate modules and routines for better maintainability, a practice that is even more vital in today's complex software projects.
On first glance we can see the code is split into modular pieces with sub routines that are grouped by feature.
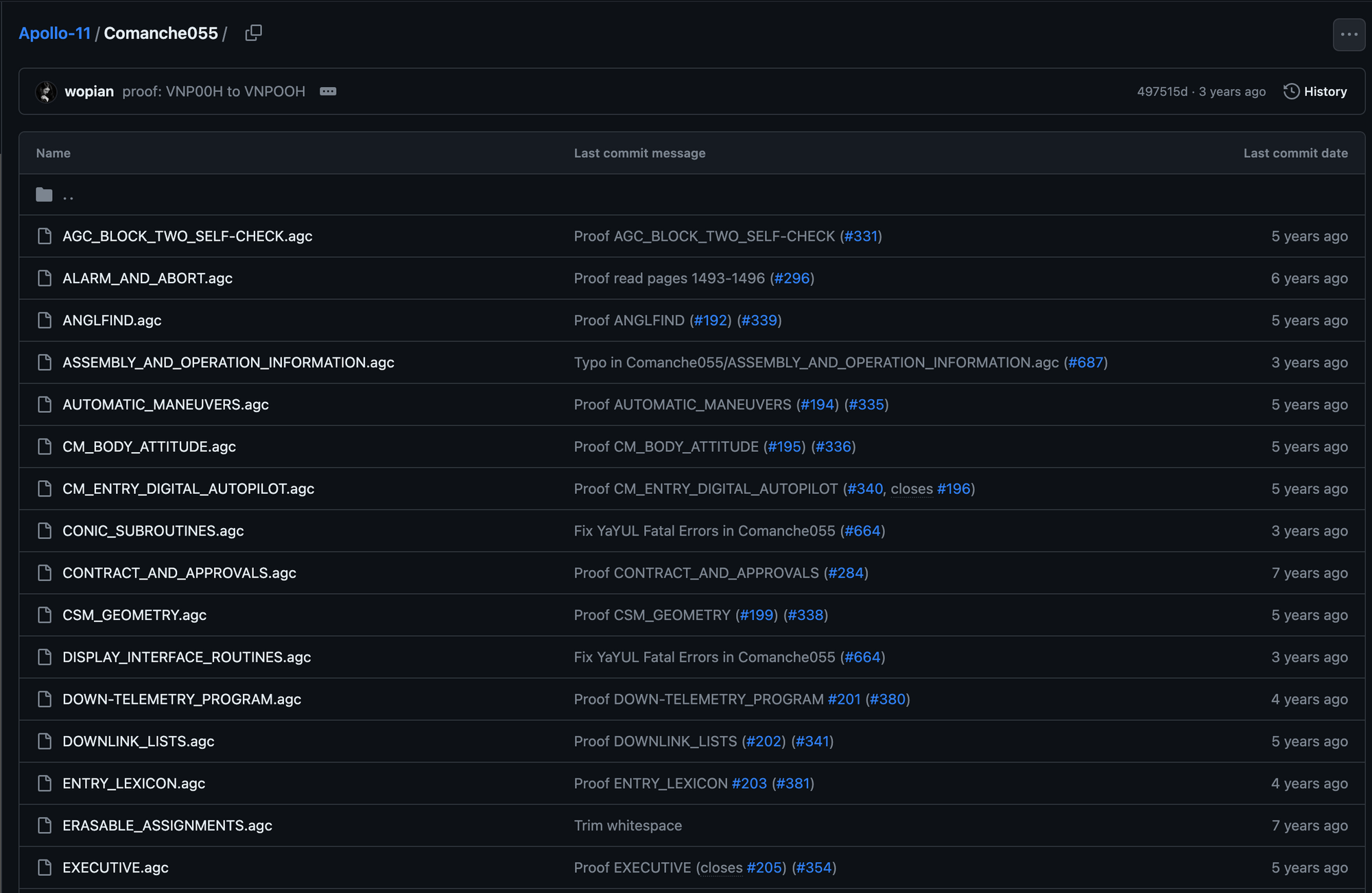
The AGC code was divided into various modules, each with its specific task. For instance, the landing procedure was divided into multiple routines, each dealing with a particular phase of the process. Each routine, given a descriptive name, had a specific role within the broader system. For example:
# *************************************
# P63: THE LUNAR LANDING, BRAKING PHASE
# *************************************
COUNT* $$/P63
P63LM TC PHASCHNG
OCT 04024
TC BANKCALL # DO IMU STATUS CHECK ROUTINE R02
CADR R02BOTH
CAF P63ADRES # INITIALIZE WHICH FOR BURNBABY
TS WHICH
CAF DPSTHRSH # INITIALIZE DVMON
TS DVTHRUSH
CAF FOUR
TS DVCNTR
CS ONE # INITIALIZE WCHPHASE AND FLPASS0
TS WCHPHASE
CA ZERO
TS FLPASS0
CS BIT14
EXTEND
WAND CHAN12 # REMOVE TRACK-ENABLE DISCRETE.
This approach underscores the value of modular programming and code organisation, making the codebase easier to understand, maintain, and update.
User-Centered Design
The AGC software was designed with user needs in mind. For instance, astronauts had to interact with the AGC through the DSKY interface, and great care was taken to ensure that the system was user-friendly and responded accurately to inputs. This focus on the user experience is a crucial aspect of modern software design.
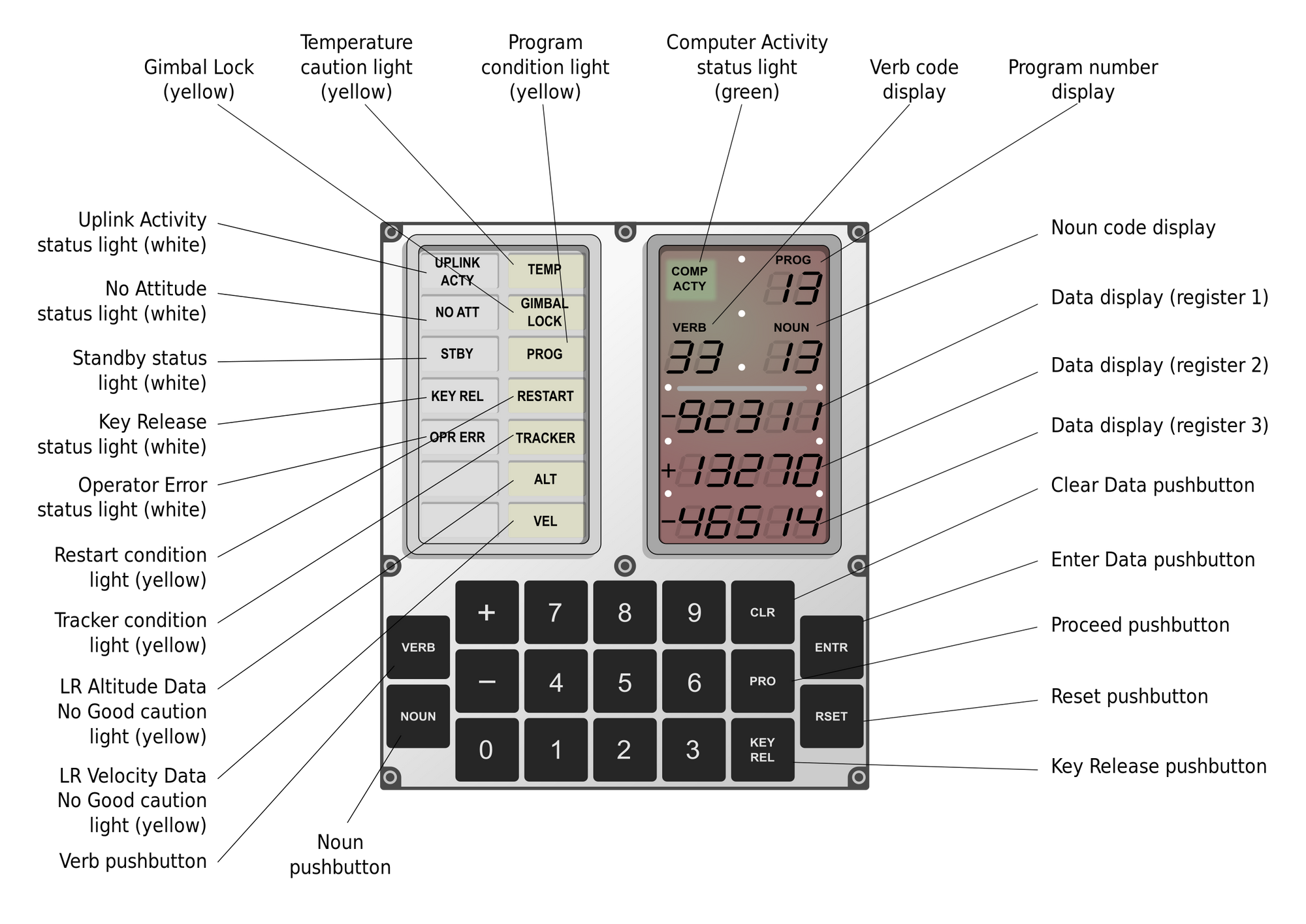
Necessity Breeds Efficiency: The Art of Simplicity
Due to the AGC's memory constraints, the code had to be incredibly efficient. This forced programmers to embrace the art of simplicity, writing lean, resourceful code that made the most out of the available resources. The computer was 1 cubic foot with only 36k WORDs of memory (smaller than an average email you'd send to your friend).
Comments were used not only as explanations but as integral parts of the narrative within the codebase, conveying important information succinctly:
BZF P63SPOT4 # BRANCH IF ANTENNA ALREADY IN POSITION 1
CAF CODE500 # ASTRONAUT: PLEASE CRANK THE
TC BANKCALL # SILLY THING AROUND
CADR GOPERF1
TCF GOTOPOOH # TERMINATE
TCF P63SPOT3 # PROCEED SEE IF HE'S LYING
P63SPOT4 TC BANKCALL # ENTER INITIALIZE LANDING RADAR
CADR SETPOS1
TC POSTJUMP # OFF TO SEE THE WIZARD...
CADR BURNBABY
The principle of simplicity is a lesson for contemporary software engineers to avoid over-complication and aim for maximum efficiency.
AGC code is filled with examples of efficient, straightforward solutions, like this piece of code for computing square roots:
SQRT TC SQRTSUB # TAKE THE SQUARE ROOT OF MPAC.
CCS MPTEMP # RETURNED NORMALIZED SQUARE ROOT. SEE IF
TCF +2 # ANY UN-NORMALIZATION REQUIRED AND EXIT
TCF DANZIG # IF NOT.
AD NEG12 # A RIGHT SHIFT OF MORE THAN 13 COULD BE
EXTEND # REQUIRED IF INPUT WAS ZERO IN MPAC,+1.
BZMF SQRTSHFT # GOES HERE IN MOST CASES.
ZL # IF A LONG SHIFT IS REQUIRED, GO TO
LXCH ADDRWD # GENERAL RIGHT SHIFT ROUTINES.
TCF GENSCR +4 # ADDRWD WAS ZERO TO PREVENT ROUND.
Robustness: Preparing for the Unknown
The AGC software was designed with a robustness that prepared it for the myriad unknowns of space flight. It was designed to prioritise vital calculations and abort less important tasks if computing power ran short. For example, the DSKY interface routine was prepared to handle any overflow conditions
This routine would activate in an overflow situation, ensuring that the AGC prioritised critical tasks and avoided a total system crash. In our contemporary context, this principle serves as a reminder to design software that can withstand unexpected situations and prioritise tasks efficiently.
The Famous 1202 Error While Descending
During the descent of the Apollo 11 Lunar Module "Eagle", a series of unexpected computer alarms occurred, famously known as "1201" and "1202" alarms. These were, essentially, "executive overflow" errors, meaning that the Apollo Guidance Computer (AGC) was being asked to do too many things simultaneously and was running out of processing capability.
The computer's design was based on an "asynchronous executive" scheduling system, which means that tasks were assigned certain priorities. In the case of Apollo 11, the high priority tasks were related to guidance and control, and the lower priority tasks involved updating the display and keyboard interface (known as the DSKY).
What happened during Apollo 11's descent was that an unnecessary radar (the Rendezvous Radar) was left on. This radar was sending unnecessary data to the computer, making it reach its processing limit, which caused the 1201 and 1202 alarms.
Here's a pseudo-code example of how the overflow condition would have been triggered:
if processing_capacity <= 0:
trigger_alarm(1201)
Despite the alarms, the mission proceeded successfully because of the AGC's robust design. The executive overflow didn't halt the entire system but only restarted the running tasks. Essential navigation and control tasks, given higher priority, continued to function correctly. The 1201 and 1202 alarms signaled to the astronauts and ground control that some tasks were being dropped, but it didn't endanger the mission as the dropped tasks were of lower priority.
Margaret Hamilton, one of the lead software engineers for Apollo, had insisted on this error detection and recovery mechanism. When these alarms occurred, the recovery mechanism was initiated, allowing the mission to proceed. Ground Control quickly recognised the error code, understood that the critical landing tasks were unaffected, and gave the astronauts a 'GO' to proceed, leading to the historic moon landing.
Commenting and Documentation
The AGC code had extensive comments to explain the purpose and functionality of the code, such as:
# THE FOLLOWING ROUTINE TORQUES THE IRIGS ACCORDING TO DOUBLE PRECISION INPUTS IN THE SIX REGISTERS
# BEGINNING AT THE ECADR ARRIVING IN A. THE MINIMUM SIZE OF ANY PULSE TRAIN IS 16 PULSES (.25 CDU COUNTS). THE
# UNSENT PORTION OF THE COMMAND IS LEFT INTACT IN THE INPUT COMMAND REGISTERS.
EBANK= 1400 # VARIABLE, ACTUALLY.
IMUPULSE TS MPAC +5 # SAVE ARRIVING ECADR.
TC CAGETSTJ # DONT PROCEED IF IMU BEING CAGED.
CCS LGYRO # SEE IF GYROS BUSY.
TC GYROBUSY # SLEEP.
And sometimes, these comments also included humour 😄:
# TEMPORARY, I HOPE HOPE HOPE
Observability
Monitoring and alarms were baked into the system. The guidance computer would periodically send telemetry about the system state back to earth and people in Houston would monitor and analyse this telemetry and make sure everything was under control.
# THE FOLLOWING CODING (FROM SNAPLOOP TO SNAPEND)IS FOR THE PURPOSE OF TAKING A SNAPSHOT OF 12 DP REGISTERS.
# THIS IS DONE BY SAVING 11 DP REGISTERS IN DNTMBUFF AND SENDING THE FIRST DP WORD IMMEDIATELY.
# THE SNAPSHOT PROCESSING IS THE MOST TIME CONSUMING AND THEREFORE THE CODING AND LIST STRUCTURE WERE DESIGNED
# TO MINIMIZE TIME. THE TIME OPTIMIZATION RESULTS IN RULES UNIQUE TO THE SNAPSHOT PORTION OF THE DOWNLIST.
# THESE RULES ARE......
# 1. ONLY 1DNADR'S CAN APPEAR IN THE SNAPSHOT SUBLIST
# 2. THE 1DNADR'S CANNOT REFER TO THE FIRST LOCATION IN ANY BANK.
SNAPLOOP TS EBANK # SET EBANK
MASK LOW8 # ISOLATE RELATIVE ADDRESS
EXTEND
INDEX A
EBANK= 1401
DCA 1401 # PICK UP 2 SNAPSHOT WORDS.
EBANK= DNTMBUFF
INDEX TMINDEX
DXCH DNTMBUFF # STORE 2 SNAPSHOT WORDS IN BUFFER
INCR TMINDEX # SET BUFFER INDEX FOR NEXT 2 WORDS.
INCR TMINDEX
SNAPAGN INCR SUBLIST # SET POINTER TO NEXT 2 WORDS OF SNAPSHOT
INDEX SUBLIST
0 0 # = CA SSSS (SSSS = NEXT ENTRY IN SUBLIST)
CCS A # TEST FOR LAST TWO WORDS OF SNAPSHOT.
TCF SNAPLOOP # NOT LAST TWO.
Code Review and Testing
While there aren't specific lines of code that demonstrate testing and review, the entire AGC codebase's correctness was critical for the mission's success. The process of writing and reviewing the code was rigorous, iterative, and exhaustive, involving extensive simulation and testing.
Use of Constants
Constants were used throughout the AGC codebase to improve readability and minimise errors. Here's an example:
# *** THE ORDER OF THE FOLLOWING SIX CONSTANTS MUST NOT BE CHANGED ***
FDPS 2DEC 4.3670 B-7 # 9817.5 LBS FORCE IN NEWTONS
MDOTDPS 2DEC 0.1480 B-3 # 32.62 LBS/SEC IN KGS/CS
DTDECAY 2DEC -38
FAPS 2DEC 1.5569 B-7 # 3500 LBS FORCE IN NEWTONS
MDOTAPS 2DEC 0.05135 B-3 # 11.32 LBS/SEC IN KGS/CS
ATDECAY 2DEC -10
Consistency
Consistent Naming Conventions: The AGC code used clear, descriptive names for variables and routines. It was evident what each variable represented or what each function did just by looking at its name. This consistency in naming made the code easier to read and understand.
Consistent Formatting and Indentation: The code was consistently formatted and indented. Consistent code formatting improves readability and can help developers understand the code more quickly and accurately. While there were no specific rules or style guides for assembly language formatting at the time, the AGC code was still structured and formatted in a consistent manner.
Consistent Commenting: The AGC code had extensive comments explaining the functionality of different parts of the code. Each subroutine was prefaced by a block comment describing its purpose, and many individual lines of code had inline comments explaining their function. This consistency in commenting practice made the AGC code more understandable and maintainable.
Consistent Error Handling: The AGC software was designed with consistent error handling routines. For example, the priority alarm displays were designed to show the most urgent alarms first, while less urgent alarms were displayed if no higher-priority alarms were active. This approach allowed for consistent handling and display of different alarm conditions.
The AGC code's adherence to consistency in these areas served as an exemplar for software engineering projects that followed. It shows that even in a high-stakes, innovative project like the Apollo missions, sticking to basic principles like consistency can contribute greatly to the success of the project.
Collaborative Development
The AGC codebase was developed by a team and shows signs of collaborative development. Although it's hard to demonstrate this with code, the comments in the code show clear communication among team members, which is key in collaborative environments. Also, the detailed documentation in the form of comments indicates that the code was written with other developers in mind.
The Apollo Guidance Computer (AGC) software was the result of the work of many talented engineers and programmers. Some of the most prominent figures involved in its development were:
Margaret Hamilton
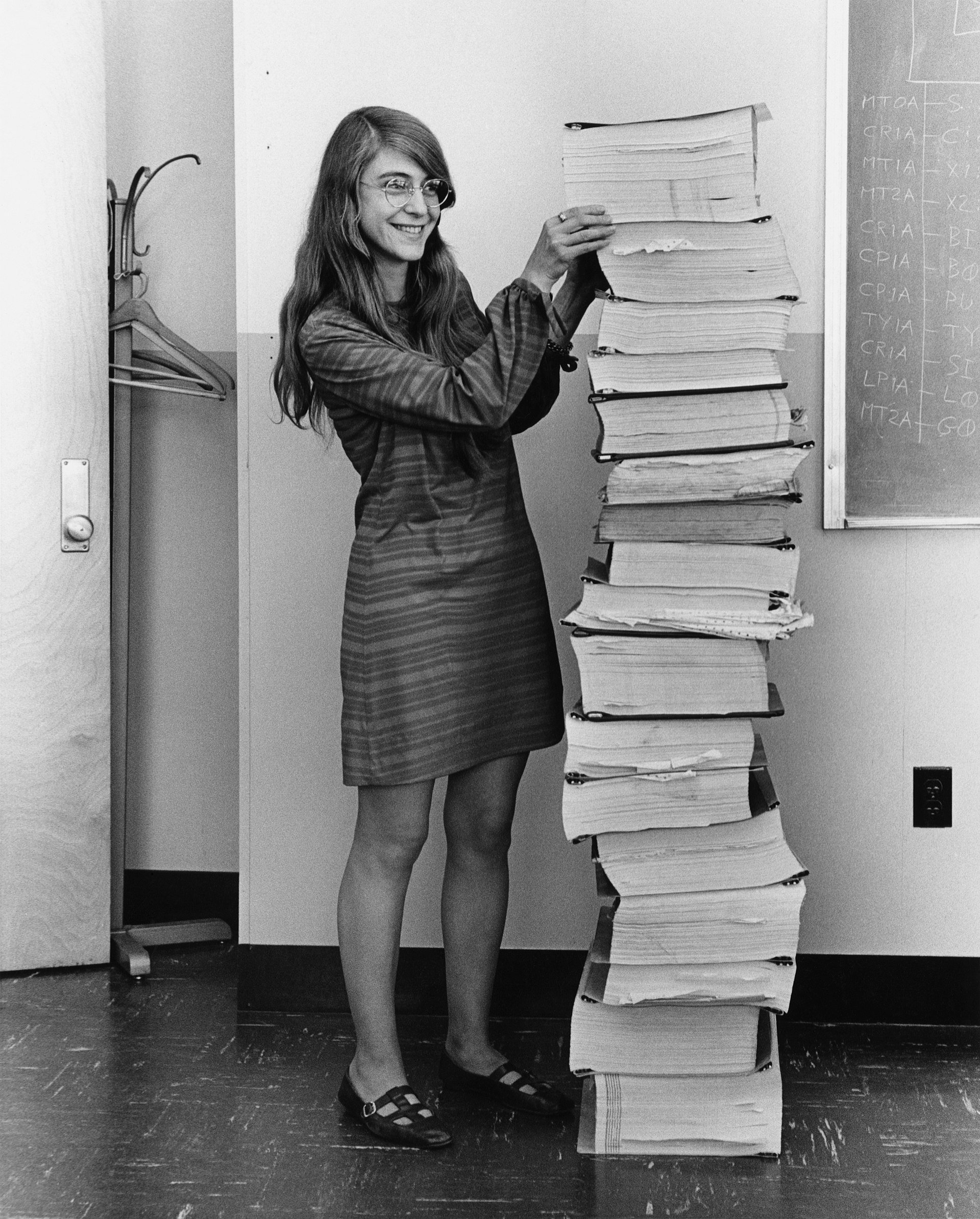
Margaret Hamilton was the Director of the Software Engineering Division of the MIT Instrumentation Laboratory, which developed on-board flight software for the Apollo space program. Her contributions were instrumental in the success of landing astronauts on the moon in 1969. Hamilton's work coined the term "software engineering," and she led the team that created the in-flight software.
Hal Laning
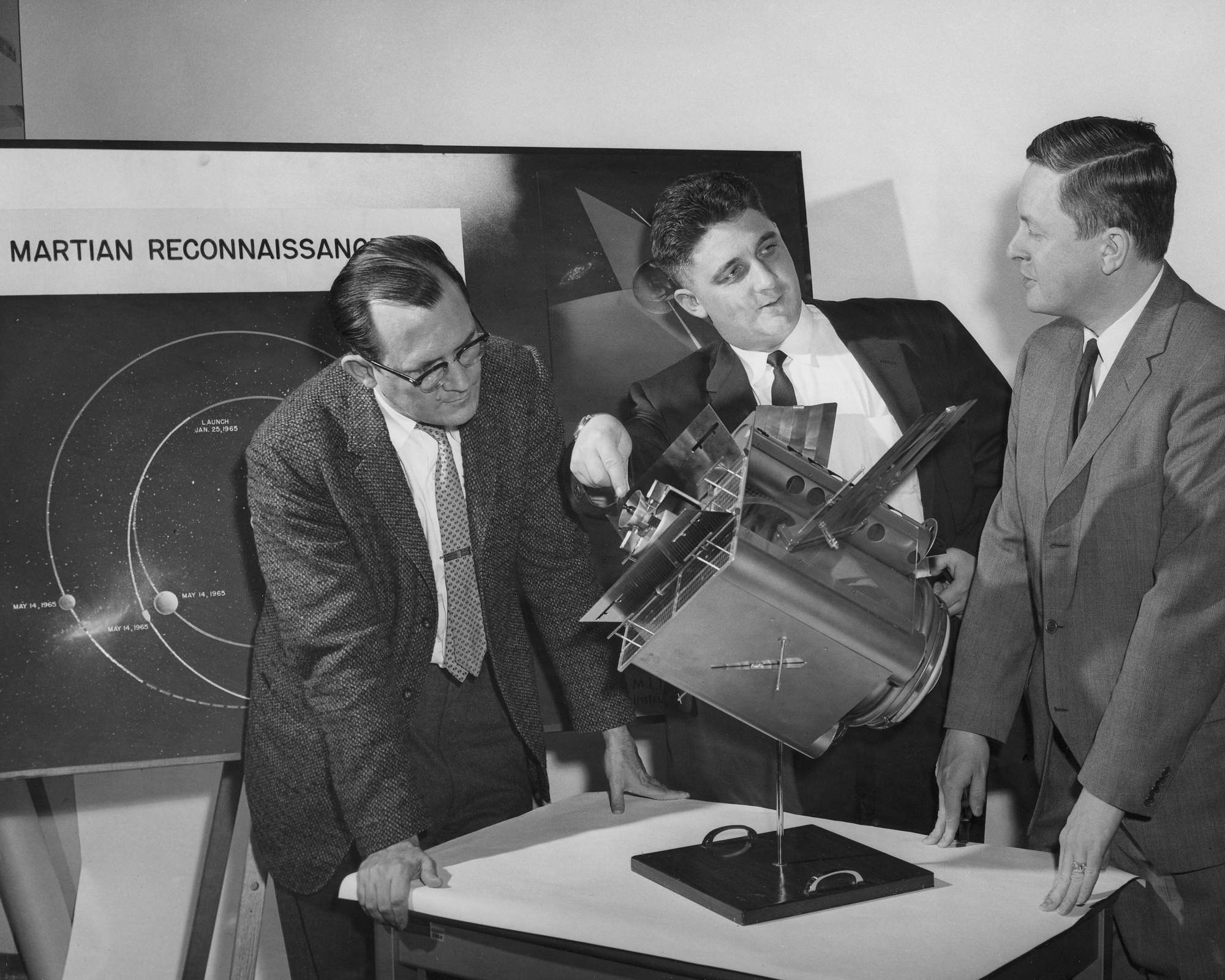
J. Halcombe "Hal" Laning developed the "Laning and Zierler System" for symbolic mathematical computation and designed the executive control system of the Apollo Guidance Computer. His work helped allow the computer to execute multiple tasks at once, prioritising more crucial tasks and deprioritizing less critical ones.
Don Eyles
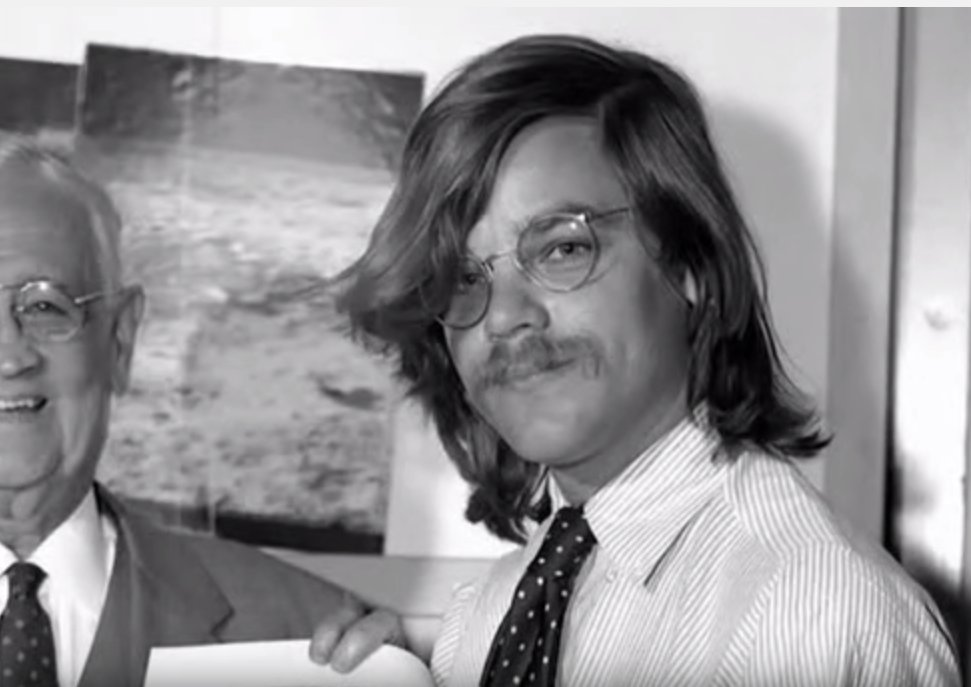
Don Eyles was a key contributor to the Apollo project, specifically to the software for the lunar module. He was responsible for programming the routines used for landing the lunar module on the moon's surface. When the infamous 1202 and 1201 alarms occurred during Apollo 11's lunar descent, it was Eyles' code that helped save the mission.
Fred Martin
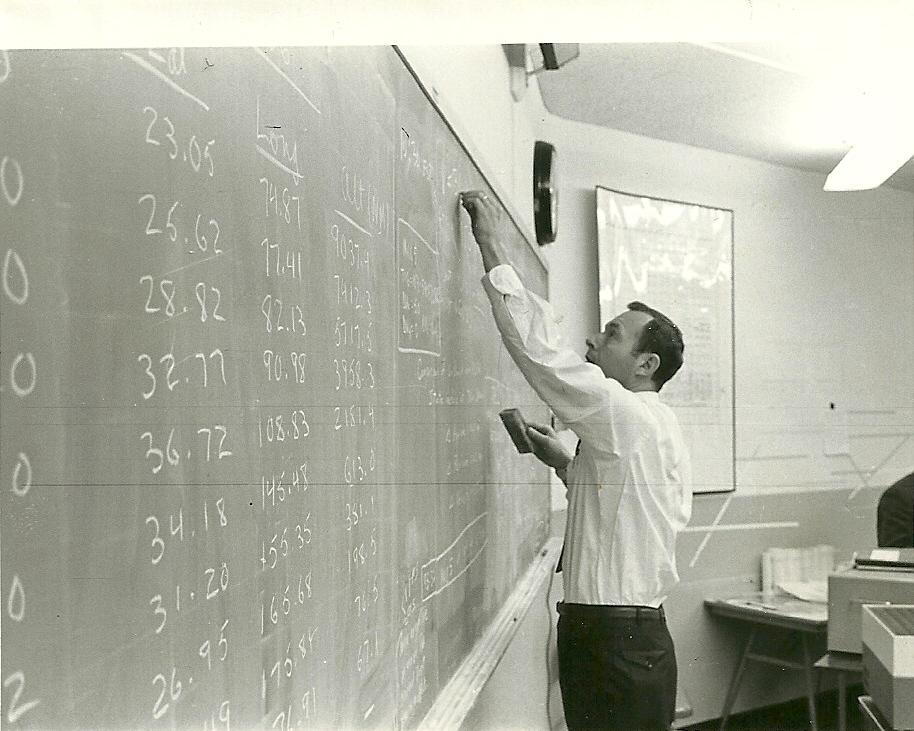
Fred Martin was responsible for the AGC's operating system. His work was crucial in developing the software routines that managed and coordinated the computer's tasks.
Dan Lickly
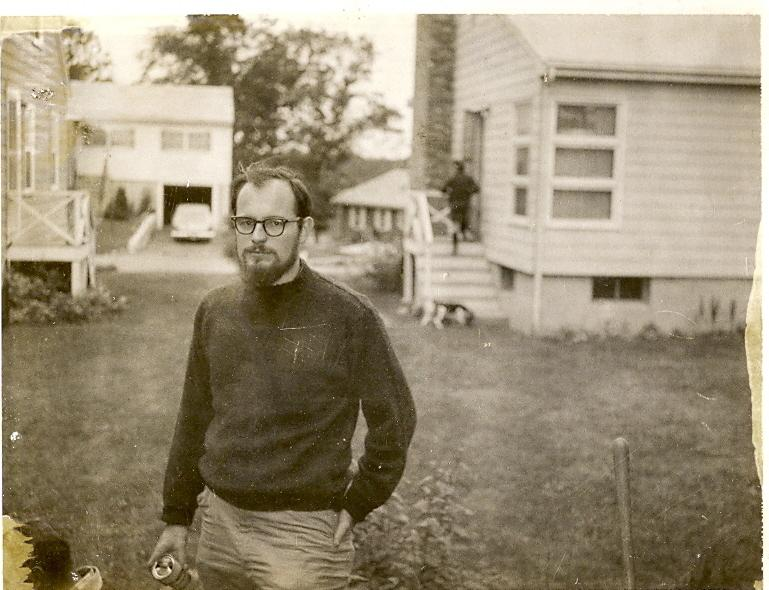
As the supervisor of the team in charge of navigation and guidance, Lickly played a critical role in developing software for the Apollo missions.
All these engineers and so many others worked as part of a large and dedicated team to develop the software for the Apollo missions. Their collaborative effort was critical in making the moon landings possible, and their work continues to influence the field of software engineering to this day.
Conclusion
Although we have seen leaps and bounds in technological advancement since the Apollo 11 mission, the principles gleaned from its 50-year-old codebase remain as valuable as ever. The AGC's assembly language code is a testament to the power of organised, simple, and robust software engineering. As we design software for the next frontier - whether it's on Earth or beyond - these principles continue to guide us towards success.
Thanks for Reading :)
Happy Engineering 🍋